Scriplet API¶
ViCANdo can be extended with custom functionality, in the form of Scriptlets. Script components are written in JavaScript and a Scriptlet can be started on demand, or by a Trigger, configured to start a Scriptlet.
You can run a script from Tools menu in ViCANdo:
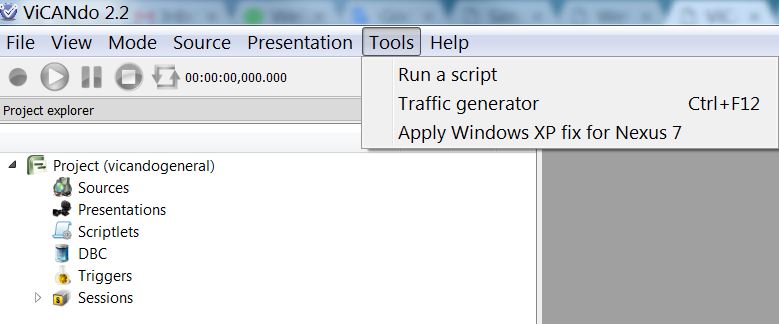
or
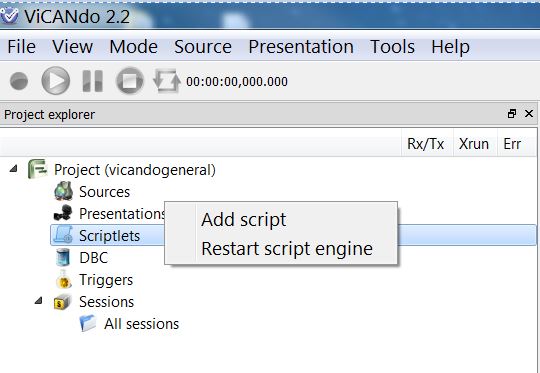
This section lists the Scriplet API functions you can use to make your own script.
Global objects¶
The global objects in ViCANdo project are:
Name |
Description |
---|---|
project |
Access project resources |
sources[] |
Array of sources avaliable in the project |
presenter[] |
Array of presenters available in the project |
scriptlet[] |
Array of Scriptlet available in the project |
trigger[] |
Array of triggers available in the project |
dbc[] |
Array of DBCs available in the project |
Global constants¶
constants:
var Idle = 0x00 // Project is idle var Preparing = 0x01 // waiting for enabled sources to be activated var Armed = 0x02 // All enabled sources are now active var Recording = 0x03 // Recording data from all enabled sources into a new session var Playing = 0x04 // Playing a previously recorded session var Pause = 0x05 // Playback or record has been paused var Rewind = 0x06 // Rewind will happen when a session has been played to its end point. It will start from the beginning on next play
Global functions¶
Prototype |
Description |
---|---|
delay(time_in_ms) |
Wait for specified time in milli-seconds |
includeScript(relative_script_path) |
Include and parse another script file into this script context |
logMessage(string message) |
Log a text message to the project console window in ViCANdo |
-
delay
(time_in_ms)¶ - Arguments
time_in_ms (int) – Time to wait in milli-seconds
-
includeScript
(relative_script_path)¶ - Arguments
relative_script_path (string) – Another script file to be included into this script context
The includeScript function provides the possibility to include another script file into the current script context. Now, it’s not pre-processor inclusion, the script will be evaluated in the current context, the variable will depend from where includeScript is called. A script will only be included once, if includeScript is called more than one time including the same script file, it will have no effect. For example:
in hello.js file:
// hello.js
project.log(“Hello World from hello.js”)
IncludeScript(“hello_inc.js”)
// Call function defined in hello_inc.js
Hello()
in hello_inc.js file:
//hello_inc.js
project.log(“Hello from hello_inc.js”)
// define a callable function
this. Hello – function {} {
project.log(“Hello from hello{} function”}
}
Project object¶
Resources¶
All the resources/components inside the project can be accessed in JavaScript:
Name |
Description |
---|---|
project.sources[] |
Array of sources available in project. Also available as a Global objects. |
project.presenter[] |
Array of presenters available in project. Also available as a Global objects. |
project.scriptlet[] |
Array of Scriptlet available in project. Also available as a Global objects. |
project.trigger[] |
Array of triggers available in project. Also available as a Global objects. |
project.dbc[] |
Array of DBC’s available in project. Also available as a Global objects. |
Every component in the project tree (Source, Presenters, Scriptlets, etc.) can be named with an Object name. This is done through the Component Properties pane in ViCANdo.
A component can be accessed from JavaScript by its object name, via the project object: project.<Object-name>.
Example using a CAN Source where its Object name is set to main_can_source:
// Send an extended CAN frame
project.main_can_source.send(0x2500,new Array(10,20,30,40,50,60,70,80)}
Methods¶
All the methods of the ViCANdo project can be accessed, for example: project.log(“This is a log”). Below is a list of all the methods.
Log a text message to the project console (just a short alias to logMessage function):
-
log
(message)¶ - Arguments
message (string) – The text message to be logged
-
currentState
()¶ - Returns
Return the current state, may be Global constants
Wait for State Change within a specified time period:
-
waitForStateChange
(timeout_in_ms)¶ - Throws
timeout exception if no state change happen in specified timeout
-
registerStateChangeCallback
(callback)¶ - Arguments
callback – The function callback that will be invoked when the project state has changed
This function registered will only be called when the script is running. After script has finished, no more callbacks are received. The callback function has two parameters: previous_state and new_state that will be some of the Global constants
Example on how to use the registerStateChangeCallback function:
project.registerStateChangeCallback{function(previous_state,new_state) {
project.log(“Previous state” + previous_state)
project.log(“New state” + new_state)
})
project.log(“waiting for state change”)
project.waitForStatechange(5000)
Activate all enabled sources, next state will be Armed:
-
activate
()¶
Start recording. Can only be done in Armed state:
-
startRecord
()¶
Stop all activity and go to Idle state:
-
stop
()¶
Clear the ViCANdo text console:
-
clearConsole
()¶
Store a value within the project:
-
storeValue
(String key, value, persistent)¶ - Arguments
key (string) – The index to the vlaue stored
value – The value to be stored. Only string values can be permanently stored.
persistent (bool) – If persistent is true, the value will be permanently stored within the project. Otherwise, the value will be permanent until the project is closed or the script engine is restarted.
Remove a stored value:
-
removeValue
(string key)¶ - Arguments
key (string) – The index to the vlaue to be removed
Retrieve a stored value from the list:
-
value
(string key)¶ - Arguments
key (string) – The index to the vlaue in the stored value list
- Returns
The value retrieved
Remove all stored values:
-
clearStoredValues
()¶
QML Presenter object¶
Set a property with given value in the QML component:
-
setProperty
(string property_name, object value)¶ - Arguments
property_name (string) – The name of the property
value (object) – The value to be set
Get the value for a specified property in the QML components:
-
property
(string property_name)¶ - Arguments
property_name (string) – The name of the property
- Returns
The value of the property
Timer object¶
-
class
Timer
()¶
Prototype |
Description |
---|---|
integer elapsedInMs() |
Elapsed time in milli-seconds since the timer was started |
integer elapsedInUs() |
Elapsed time in micro-seconds since the timer was started |
restart() |
Restart the timer |
-
elapsedInMs
()¶ - Returns
Elapsed time in milli-seconds
-
elapsedInUs
()¶ - Returns
Elapsed time in micro-seconds
-
restart
()¶
Example:
var timer1 = Timer()
// do something...
var elapsed_time_in_us = timer1.elapsedInUs()
var elapsed_time_in_ms = timer1.elapsedInMs()
Timer with a callback¶
Prototype |
Description |
---|---|
Timer(<JavaScript_function>) |
Create a new timer with a given JavaScript function that will be invoked on timeout |
start(timeout_in_ms) |
Start timer with specified timeout in milli-seconds |
stop() |
Stop the timer |
singleshot = true/false |
Single shot property, set to true and timer will only expire 1 time after start() |
-
class
Timer
(JavaScript_function)¶ - Arguments
JavaScript_function (string) – The JavaScript function that will be invoked on timeout
-
start
(timeout_in_ms)¶ - Arguments
timeout_in_ms – Timeout in milli-seconds
-
stop
()¶ Note
All timers will be automatically stopped after script has finished
Example using a periodic timer:
var counter = 0
var t1 = new Timer(function{}{
project.log(“Timer callback” + counter)
counter ++
})
T1.start(1000)
Example using a ingle-shot timer:
var t1 = new Timer(function{} {
project.log (“Timer has expired”)
})
T1.singleshot = true
T1.start(1000)
System functions¶
Provides a collection of system functions.
Start a new program with the arguments given in argument list and wait for the process to finish. If the script engine is stopped before the program has finished the process will be brutally terminated. The return value from this function contains the process exit code the standard and error output as a string in a array as {<exit_code>,<standard_output>,< Standard-error>}:
-
system.
executeProgram
(string program, argument_list)¶ - Arguments
program (string) – The program to be executed
argument_list – The arguments when executing with the program
- Returns
array of status: [<exit_code>,<standard_output>,< Standard-error>]
Start a new process program with the arguments given in arguments list and spawn it in the background. The return result is the PID of the process:
-
system.
executeProgramDetached
(string program, argument_list)¶ - Arguments
program (string) – The program to be executed
argument_list – The arguments when executing with the program
- Returns
PID of the process
List all available text codecs by name. Return an array of string objects:
-
system.
availableTextCodecs
()¶
File System functions¶
Provides a collection of functions for file I/O and basic file-system manipulation. All functions are provides by the fs object:
fs object¶
Remove/delete a give path on file-system:
-
fs.
unlink
(string path)¶ - Arguments
path (string) – Path to be deleted
Rename file specified by old_path to new_path:
-
fs.
rename
(string old_path, string new_path)¶ - Arguments
old_path (string) – The old path of the file
new_path (string) – The new path of the file
Return true if the specified path exists on the file-system:
-
fs.
fileExists
(string path)¶ - Arguments
path (string) – The path to be checked
- Returns
true if path exists, false if path doesn’t exist
Return true if the specified path is a directory:
-
fs.
openDirectory
(string path)¶ - Arguments
path (string) – The path given
- Returns
true if the path is a directory, false otherwise
Open a file. Mode can be “r’ for read only.”w” only write, ”rw” for read and write, “a” for append stream object:
-
fs.
openFile
(string path, string mode)¶ - Arguments
path (string) – The path of the file
mode (string) – The open file mode. “r” for read only. “w” for wirte only. “rw” for read and wirte. “a” for append stream object.
- Returns
The file stream object
Close an opened stream:
-
fs.
close
(stream stream)¶ - Arguments
stream (stream) – The stream to be closed
Returns the absolute path of the process current working directory:
-
fs.
currentDirectory
()¶ - Returns
The path of the process current wokring directory
Returns the absolute path of the user’s home directory. This will differ depending on operating system:
-
fs.
homeDirectory
()¶ - Returns
The path of the user’s home directory
Returns the absolute path of the operating system’s temporary directory:
-
fs.
tempDirectory
()¶ - Returns
The path of the operating system’s temporary directory
Returns directory separator used on the target system, “I “under Unix (including Mac OS X) and “” under Windows:
-
fs.
separator
()¶ - Returns
The separator: “I “under Unix (including Mac OS X) and “” under Windows
Change the current directory to path:
-
fs.
chdir
(string path)¶ - Arguments
path (string) – Path to be changed to
Create directory path:
-
fs.
mkdir
(string path)¶ - Arguments
path (string) – Path to be created. The path should be relative to currentDirectory().
Remove a directory:
-
fs.
rmdir
(string path)¶ - Arguments
path (string) – Path to be removed, must be a directory and it is relative to currentDirectory().
Returns a list of files in the specified path:
-
fs.
list
(string path)¶ - Arguments
path (string) – The specified path
- Returns
a array of files in the specified path. e.g. [“file1”, “file2”, “file3”]
Returns a list of files and directories in the specified path:
-
fs.
listFiles
(string path)¶ - Arguments
path (string) – The specified path
- Returns
a array of files and directories in the specified path.
Return a fileinfo object for the given path. The fileinfo object provides system independent file information:
-
fs.
fileInfo
(string path)¶ - Arguments
path (string) – The given path
- Returns
stream object¶
Write a line to stream, LF is added to the end:
-
stream.
writeLine
(string string)¶ - Arguments
string (string) – The string to be written
Read a line from the stream. If not a complete line is read from in timeout_in_ms millis-seconds, execution is aborted with an exception:
-
stream.
readLine
(timeout_in_ms)¶ - Arguments
timeout_in_ms – The timeout time in milli-seconds
- Returns
The line was read
- Throws
exception – If not a complete line is read from when reach the timeout_in_ms millis-seconds, execution is aborted with an exception
Flushes any buffered data to the stream:
-
stream.
flush
()¶
Return true if at end of file:
-
stream.
eof
()¶
Set the text codec to used when read and writing text from this stream. Use system.availableTextCodecs() list available text codecs:
-
setEncoding
(string codec_name)¶ - Arguments
codec_name (string) – The name of the codec.
fileinfo object¶
A fileinfo object contains below properties:
Property |
Description |
---|---|
path |
Absolute path including the file name |
created |
The date and time when the file was created |
lastModified |
The date and time when the file was last modified |
lastRead |
The date and time when the file was last read (accessed) |
size |
The size of the file in bytes |
owner |
The Owner of the file. On systems where files do not have owners or if an error occurs. It will contain an empty string |
group |
The group of the file. On windows on systems where files do not have owners or if an error occurs it will contain an empty string |
directory |
Set to true if this object points to a directory or to a symbolic link to a directory. Otherwise set to false |
executable |
Set to true if the user can read the file. Otherwise set to false |
hidden |
Set to true if this is a ‘hidden’ file. Otherwise set to false |
readable |
Set to true if the user can read the file. Otherwise set to false |
writable |
Set to true if the user can write to the file. otherwise set to false |
symlink |
Set to true if this object points to a symbolic link(or to a shortcut on Windows). Otherwise set to false |
Example creating a text file and writing some lines:
var out = fs.openFile("/tmp/test.txt","w")
out.writeLine("Test line 1")
out.writeLine("Test line 2")
out.writeLine("Test line 3")
out.writeLine("Test line 4")
fs.close(out)
Example reading some lines from a text file:
var f = fs.openFile("/tmp/test.txt","r")
var line_no = 1;
while (!f.eof()) {
project.logMessage("line " + line_no + " " + f.readLine());
line_no ++;
}
fs.close(f)
CAN Source object¶
Constants¶
-
can.flag.
Rtr = 0x00001
¶
-
can.flag.
Standard = 0x00002
¶
-
can.flag.
Extended = 0x00004
¶
-
can.flag.
Wakeup = 0x00008
¶
-
can.flag.
NError = 0x00010
¶
-
can.flag.
ErrorFrame = 0x00020
¶
-
can.flag.
TxMsgAcknowledge = 0x00040
¶
-
can.flag.
TxMsgRequest = 0x00080
¶
-
can.flag.
ErrorMask = 0x0ff00
¶
-
can.flag.
ErrorHWOverrun = 0x00200
¶
-
can.flag.
ErrorSWOverrun = 0x00400
¶
-
can.flag.
ErrorStuff = 0x00800
¶
-
can.flag.
ErrorForm = 0x01000
¶
-
can.flag.
ErrorCRC = 0x02000
¶
-
can.flag.
ErrorBIT0 = 0x04000
¶
-
can.flag.
ErrorBIT1 = 0x08000
¶
-
can.flag.
Statistic = 0x10000
¶
Methods¶
Send a CAN frame, extended ID is default:
-
send
(uint id, byte[] data)¶ - Arguments
id (uint) – The identifier of the CAN frame
data (byte[]) – Array of CAN frame data. The format is: [b0, b1, b2, b3, b4, b5, b6, b7]
Send an extended CAN frame:
-
sendExtended
(int id, byte[] data)¶ - Arguments
id (int) – The identifier of the CAN frame
data (byte[]) – Array of CAN frame data. The format is: [b0, b1, b2, b3, b4, b5, b6, b7]
Send a standard CAN frame:
-
sendStandard
(int id, byte[] data)¶ - Arguments
id (int) – The identifier of the CAN frame
data (byte[]) – Array of CAN frame data. The format is: [b0, b1, b2, b3, b4, b5, b6, b7]
Send a remote CAN frame, default is extended:
-
sendRemote
(int id, byte[] data)¶ - Arguments
id (int) – The identifier of the CAN frame
data (byte[]) – Array of CAN frame data. The format is: [b0, b1, b2, b3, b4, b5, b6, b7]
Send a standard remote CAN frame:
-
sendStandardRemote
(int id, byte[] data)¶ - Arguments
id (int) – The identifier of the CAN frame
data (byte[]) – Array of CAN frame data. The format is: [b0, b1, b2, b3, b4, b5, b6, b7]
Send an extended remote CAN frame:
-
sendExtendedRemote
(int id, byte[] data)¶ - Arguments
id (int) – The identifier of the CAN frame
data (byte[]) – Array of CAN frame data. The format is: [b0, b1, b2, b3, b4, b5, b6, b7]
Receive a CAN frame, if nothing is received in timeout_in_ms script execution is aborted with an exception:
-
receive
(timeout_in_ms)¶ - Arguments
timeout_in_ms – Time out time in milli-seconds
- Returns
CAN frame array in the form of: [ID, Flags, B0, B1, B2, B3, B4, B5, B6, B7]
- Throws
exception – If nothing is received in specified timeout time
Returns true if source is attached to virtual CAN interface:
-
isVirtual
()¶ - Returns
true if the source is virtual CAN interface. Otherwise false.
Properties¶
Property |
Type |
Description |
---|---|---|
deviceList |
string-array |
List of current available CAN interfaces |
device |
Integer |
The current device index |
deviceName |
string |
The name of the current CAN interface |
Example using the a CAN source object. source[0] is a CAN source:
// Send an extended CAN 29bit frame
source[0].send(0x2500,new Array(10,20,30,40,50,60,70,80));
// Send a standard CAN 11bit frame
source[0].sendStandard(0x100,new Array(10,20,30,40,50,60,70,80));
Example Recieving a CAN frame:
var can_frame;
while (true) {
can_frame = source[0].receive(5000)
project.logMessage("Received CAN frame: " + can_frame)
}
This example is waiting to recieve a CAN frame for 5000 ms. If the CAN frame is not recieved on time, ViCANdo console window will print “Error: Timed out waiting for CAN frame” In ViCANdo console window, the recieved frames are displayed like this:
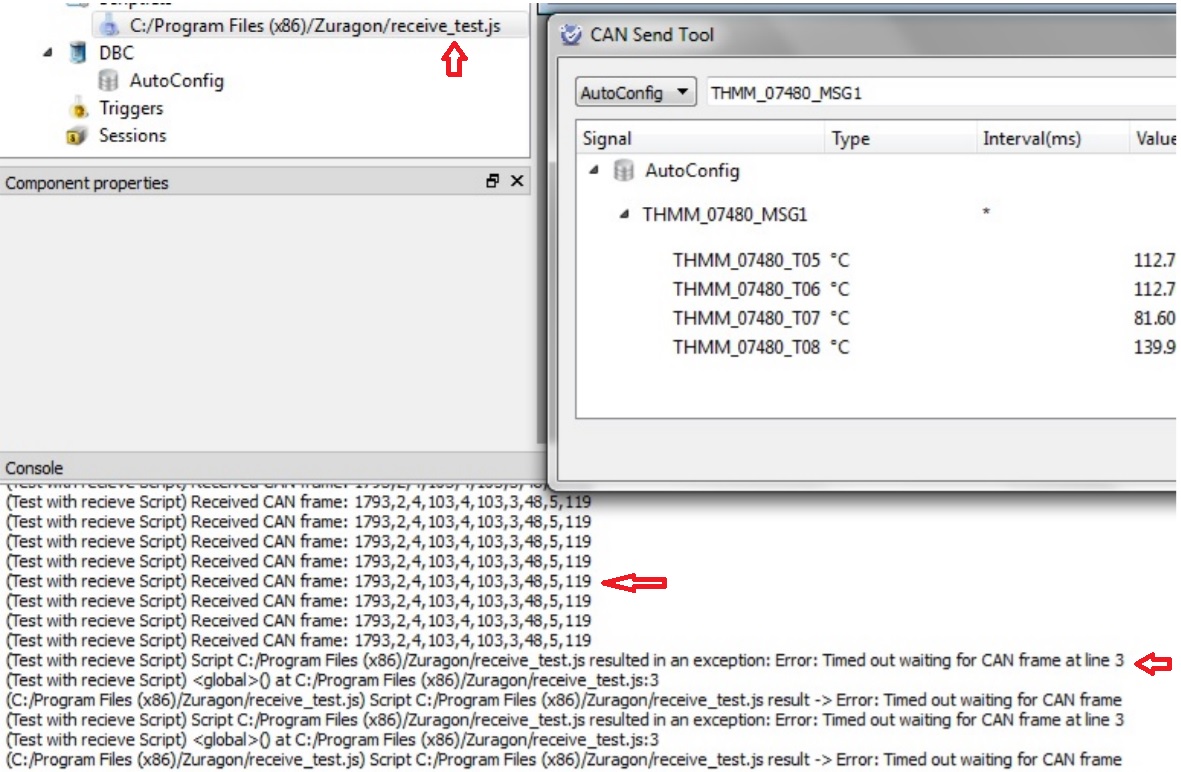
The message in the above console window
(Test with recieve Script) Received CAN frame: 1793,2,4,103,4,103,3,48,5,119
means:
(project name) Recieved CAN frame: ID, Flags, B0, B1, B2, B3, B4, B5, B6, B7
J1939 Source object¶
Send J1939 message addressed to a node in the network:
-
send
(sa, da, priority, pgn, byte[] data)¶ - Arguments
sa – Source address
da – Destination address
priority – The priority of the message
pgn – The PGN number
data (byte[]) – The data of the message
Send a broadcast J1939 message:
-
sendBAM
((sa, da, priority, pgn, byte[] data)¶ - Arguments
sa – Source address
da – Destination address
priority – The priority of the message
pgn – The PGN number
data (byte[]) – The data of the message
Receive a J1939 message, if nothing is received in timeout_in_ms, script execution is aborted with an exception:
-
receive
(timeout_in_ms)¶ - Arguments
timeout_in_ms – Timeout time in milli-seconds
- Returns
Array contains J1939 message information. The format is: [sa, da, priority, pgn flags, b0, b1, b2, b3, b4, b5…]
ISO15765 Source object¶
Basic Contepts and abbreviations¶
PCI
Protocol Control Information. May be single-Frame, First-Frame, Flow-Control or Consecutive-Frame. Find more details in the ISO15765 specification.
Data
The payload of an ISO15765 message. Find more details in the ISO15765 specification.
M Type
May be diagnostics or remote diagnostics. The parameter Mtype shall be used to identify the type and range of address information parameters included in a service call. This part of ISO 15765 specifies a range of two values for this parameter. The intention is that users of the documents can extended the range of values by specifying other types and combination of address information parameter to be used with the network layer protocol specified in this document. For each such new range of address information a new value for the Mtype parameter shall be specified to identify the new address information.
If Mtype = diagnostics then the address information AI shall consist of the parameters SA,TA and TAtype
If Mtype = remote diagnostics then the parameters AI shall consist of the parameters SA, TA, TAtype and AE
AI
These parameters refers to addressing information. As a whole, the AI parameters are used to identify the source address (SA), target address (TA) of message senders and recipients as well as the communication model for the message (TAType) and the optional address extension (AE).
SA
Network Source Address, 1 byte unsigned integer value range 00-FF hex. The SA parameter shall be used to encode the sending network layers protocol entity.
TA
Network Target Address, 1 byte unsigned integer value, range 00-FF hex. The TA parameter shall be used to encode the receiving network layer protocol entity.
TAType
Network Target Address type physical or functional. Physical addressing (1-1 communication) shall be supported.
AE
Network Address Extension, 1 byte unsigned integer value, range 00-FF Hex. The AE parameter is used to extend the available address range for large networks, and tonencode both sending and receiving network layers entities of subnets other than the local network where the communication takes place. AE is only part of the addressing information if Mtype is set to remote diagnostics.
Addressing modes¶
Normal addressing
For each combination of SA, TA, TAtype and Mtype, a unique CAN identifier is assigned. PCI and Data is placed within the CAN frame data. For this mode an addressing map must be defined, see <iso15765-source>.setAddressMap (address_map)
Fixed addresssing
Normal fixed addressing is a sub format of normal addressing where the mapping of the address information into the CAN identifier is further defined. In the general case of normal addressing, described above, the correspondence between AI and the CAN identifier is left open. For normal fixed addressing, only 29 bit CAN identifiers are allowed.
Extended addressing
For each combination of SA, TAtype and Mtype, a unique CAN identifier is assigned. TA is placed in the first data byte of the CAN frame data. PCI and Data is placed in the remaining bytes of the CAN frame data field. For this mode an addressing map must be defined, see <iso15765-source>.setAddressMap (address_map)
Mixed addressing
Mixed addressing is the addressing format to be used if Mtype is set to remote diagnostics.
29 bit CAN identifier
The address information(AI) is in the 29 bit CAN identifier, and the first CAN frame data byte shall be the AE.
11 bit CAN identifier
For each combination of SA, TA and TAtype a unique CAN identifier is assigned. AE is placed in the first data byte of the CAN frame data. PCI and Data is placed in the remaining bytes of the CAN frame data field. For this mode an addressing map must be defined, see <iso15765-source>.setAddressMap (address_map)
Constants¶
-
tp.iso15765.
NormalAddressMode = 0x0
¶
-
tp.iso15765.
ExtendedAddressMode = 0x1
¶
-
tp.iso15765.
FixedAddressMode = 0x2
¶
-
tp.iso15765.
MixedAddressMode = 0x3
¶
-
tp.iso15765.
Physical = 0x0
¶
-
tp.iso15765.
Functional = 0x1
¶
-
tp.iso15765.
ExtAddrFlag = 0x20000
¶
-
tp.iso15765.
UnknownTypeFlag = 0x40000
¶
Methods¶
Set the address map, used for Normal, Extended and Mixed addressing (using 11-bit CAN identifiers), see example below.
-
setAddressMap
(address_map)¶ - Arguments
address_map – The address map to be set.
Send a ISO15765 message, to from SA, addressed to TA:
-
send
(sa, ta, ta_type, id, byte[] data)¶ - Arguments
sa – SA
ta – TA
ta_type – TAType
id – identifier
data (byte[]) – Data of the ISO15765 message
Send a ISO15765 message specifying the request and response CAN id. NOTE: this function can only be used when in Normal addressing mode. When sending functional or single frame messages can_response_id is allowed to be set to null:
-
sendNormalRaw
(can_request_id, can_response_id, byte[] data)¶ - Arguments
can_request_id – Request CAN identifier
can_response_id – Response CAN identifier
data (byte[]) – Data of the ISO15765 message
Receive a ISO15765 message, if nothing is received in timeout_in_ms script execution is aborted with an exception. On successful receive of a message an array is returned:
-
receive
(timeout_in_ms)¶ - Arguments
tiemout_in_ms – Timeout time in milli-seconds
- Returns
For MixedAddressMode, array format is: [<CAN-ID>,<SA>,<TA>,<TAType>,<AE>,<flags>,<data>]. For other mode, array format is: [<CAN-ID>,<SA>,<TA>,<TAType>,<flags>,<data>]
- Throws
exception – If nothing is received in specified timeout time, script exception is aborted.
Defining an address map¶
For Normal, Extended, and Mixed address mode (with 11bit CAN identifiers) an address map must be defined. A unique CAN identifier is defined for each combination of SA, TA and TAType. Note that for extended address mode only SA and TAType is used.
Example defining an address map having 3 nodes in the network, with addresses 1, 2 and 5:
var address_map = [ { id:0x242, sa:5, ta:1,ta_type:tp.iso15765.Physical },
{ id:0x243, sa:5, ta:2, ta_type:tp.iso15765.Physical },
{ id:0x542, sa:1, ta:2, ta_type:tp.iso15765.Physical },
{ id:0x543, sa:2, ta:1, ta_type:tp.iso15765.Physical },
{ id:0x643, sa:1, ta:5, ta_type:tp.iso15765.Physical },
{ id:0x843, sa:2, ta:5, ta_type:tp.iso15765.Physical } ]
project.iso_source.setAddressMap(address_map)
Example sending a Single-Frame:
// SA 1 TA 2 TAType Physical
iso_source.send(1,2, tp.iso15765.Physical, [22,23,24,25,26,27]);
Example sending a Multi-Frame:
var packet = [];
for ( i = 0; i < 33; ++i ) {
packet.push(i)
}
// SA 2 TA 5 TAType Physical
iso_source.send(2,5,tp.iso15765.Physical, packet);
LIN Source object¶
Constants¶
-
lin.flag.
ParityError = 0x00001 // Rx: parity error
¶
-
lin.flag.
ChecksumError = 0x00002 // Rx: checksum error
¶
-
lin.flag.
NoData = 0x00004 // Rx: header only
¶
-
lin.flag.
BitError = 0x00008 // Tx: transmitted 1, got 0 or vice versa
¶
-
lin.flag.
TxSlaveResponse = 0x00010 // Rx: echo of a slave response we transmitted
¶
-
lin.flag.
ClassicChecksum = 0x00020 // Rx or Tx
¶
-
lin.flag.
TxMsgAcknowledge = 0x00040 // Tx message acknowledge
¶
-
lin.flag.
TxMsgRequest = 0x00080 // Tx message request
¶
-
lin.flag.
ErrorHWOverrun = 0x00200 // Rx: LIN interface overrun
¶
-
lin.flag.
ErrorSWOverrun = 0x00400 // Rx: receive queue overrun
¶
-
lin.flag.
SynchError = 0x00800 // Synch error
¶
-
lin.flag.
WakeUp = 0x01000 // A wake up frame was received
¶
Methods¶
Send data on the LIN bus:
-
send
(uint id, byte[] data)¶ - Arguments
id (uint) – identifier
data (byte[]) – array of data to be sent. The format is: [b0, b1, b2, b3, b4, b5, b6, b7]
Receive data on the LIN bus, if nothing is received in timeout_in_ms script execution is aborted with an exception:
-
receive
(timeout_in_ms)¶ - Arguments
timeout_in_ms – Timeout time in milli-seconds
- Returns
array of received data. The format is: [id, flags, [b0,b1,b2,b3,b4,b5,b6.b7] ]
Set or update a message response data for a specified ID, only for slave:
-
setSlaveResponse
(uint id, byte[] data)¶ - Arguments
id (uint) – identifier
data (byte[]) – array of data. The format is: [b0, b1, b2, b3, b4, b5, b6, b7]
Clear a message response, only for slave:
-
clearslaveResponse
(uint id)¶ - Arguments
id (uint) – identifier
Clear all message response, only for slave:
-
clearslaveResponse
()¶
For master node, request a response from a slave:
-
sendMasterRequest
(uint id)¶ - Arguments
id (uint) – identifier
Send a wake-up frame:
-
sendWakeUp
()¶
LIN master and slave example:
var master = project.master_lin_channel
var slave = project.slave_lin_channel
slave.setSlaveResponse(10, [1,2,3,4,5,6,7,8])
slave.setSlaveResponse(11, [10,20,30,40,50,60,70,80])
slave.setSlaveResponse(12, [11,21,31,41,51,61,71,81])
slave.setSlaveResponse(13, [12,22,32,42,52,62,72,82])
slave.setSlaveResponse(14, [13,23,33,43,53,63,73,83])
slave.setSlaveResponse(15, [14,24,34,44,54,64,74,84])
project.log("Send master request")
for ( var i = 10; i <=15; ++i )
{
master.sendMasterRequest(i)
var response
do {
response = slave.receive(100)
} while( response[0] != i)
project.log("Slave response: " + response)
}